Components
Library dependency tower for xo-unit:
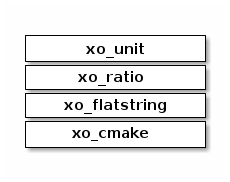
Install instructions here
Abstraction tower for xo-unit components:
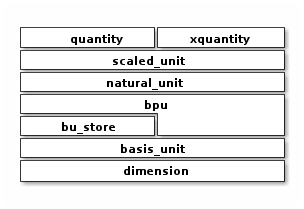
-
A quantity with unit checking and conversion done at compile-time
#include "xo/unit/quantity.hpp" auto q1 = xo::qty::qty::kilometers(7.5);
-
A quantity with unit checking and conversion done at run-time. This is useful if unit information isn’t known at compile time, for example if reading units from console input.
#include "xo/unit/xquantity.hpp" xquantity qty1(7.5, xo::qty::u::foot)
-
#include "xo/unit/scaled_unit.hpp" auto u = xo::qty::u::millimeter;
A unit involving zero or more dimensions, and associated conversion factor.
can express result of arithmetic involving multiple scales, by reporting an outer scalefactor
a scaled unit is ‘natural’ if its outer scalefactor is 1.
quantities are represented by associating a natural scaled_unit instance
scaled_units are closed under multiplication and division.
multiplication and division commit to a single
basis_unit
for each dimension.
-
#include "xo/unit/natural_unit.hpp" auto u = xo::qty::nu::millimeter;
A unit involving zero or more dimensions, and at most one scale per dimension. A quantity instance is always represented as a dimensionless multiple of a natural unit
natural_units are not closed under multiplication and division. (for example consider
xo::qty::qty::foot * xo::qty::qty::meter
)
-
A rational (usually integer) power of a basis unit. Has a single dimension.
#include "xo/unit/bpu.hpp" xo::qty::bpu(xo::qty::detail::bu::millimeter, xo::qty::power_ratio_type(2)); // mm^2
-
Associates basis units with abbreviations. Abbreviations used to decorate printed quantities.
For example
bu::kilogram
=>"kg"
#include "xo/unit/bu_store.hpp" xo::qty::bu_abbrev_store.bu_abbrev(xo::qty::detail::bu::picogram); // "pg"
-
A unit with a single dimension and scale.
#include "xo/unit/basis_unit.hpp" auto b = xo::qty::detail::bu::picogram;
-
identifies a dimension, such as mass or time.
#include "xo/unit/dimension.hpp" auto d = xo::qty::dimension::mass;
Representation
Worked example using xo::qty::quantity
.
1#include "xo/unit/quantity.hpp"
2...
3using xo::qty;
4namespace q = xo::qty::qty;
5
6// 7.55km.min^-2
7quantity qty1 = 7.55 * q::kilometer / (q::minute * q::minute);
Note: in diagrams below, components with pale blue background are discarded before runtime
representation for quantity 7.55km.min^-2
1// 123ng
2quantity qty2 = q::nanograms(123);
representation for quantity 123 nanograms
1// (123*7.55) ng.km.min^-2
2quantity qty3 = qty2 * qty1;
quantity 928.65 ng.km.min^-2
1namespace u = xo::qty::u;
2
3// (123*7.55) ng.km.min^-2 ==> 2.57958e-10kg.m.s^-2
4
5constexpr auto newton = u::kilogram * u::meter / (u::second * u::second);
6
7quantity<newton> qty3b = qty3;
8
9// quantity qty3b = qty3.rescale_ext<newton>();
quantity 928.65 ng.km.min^-2